Unity 11.11 케릭터 동적 생성하고 무기 장착및 교체하기
C#/실습 2019. 11. 11. 17:13예제:
hero가 처음에는 무기를 갖고 있다가 monster에게 넘겨준다(코드상으로만)
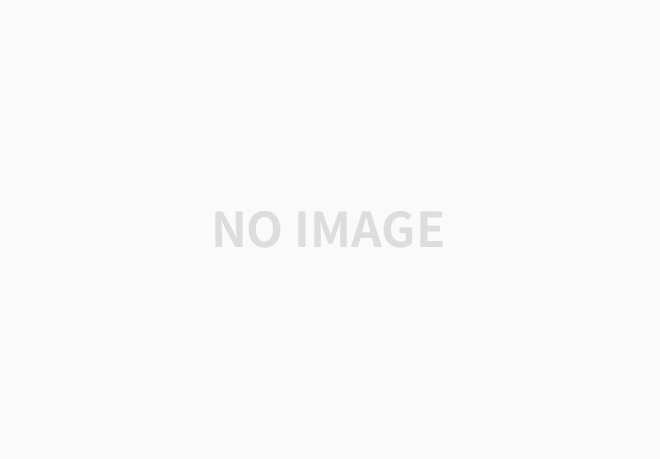
코드:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class App2 : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
GameObject heroGO = new GameObject("Hero");
heroGO.transform.position = new Vector3(-2, 0, 0);
Hero hero = heroGO.AddComponent<Hero>();
CharacterInfo info = new CharacterInfo() { id = 300, hp = 100 };
hero.info = info;
GameObject monsterGO = new GameObject("Monster");
Monster monster = monsterGO.AddComponent<Monster>();
monster.info = info;
GameObject heroModel = Instantiate(heroPrefab);
heroModel.transform.SetParent(heroGO.transform,false);
hero.model = heroModel;
GameObject dummyhandGOHero = FindChild(heroGO.transform,"DummyRHand");
GameObject monsterModel = Instantiate(monsterPrefab);
monsterModel.transform.SetParent(monsterGO.transform, false);
monster.model = monsterModel;
GameObject dummyhandGOMonster = FindChild(monsterGO.transform, "DummyRHand");
GameObject swordModel = Instantiate(swordPrefab);
swordModel.transform.SetParent(dummyhandGOHero.transform, false);
swordModel.transform.localPosition = Vector3.zero;
swordModel.transform.SetParent(dummyhandGOMonster.transform, false);
GameObject swordGO = new GameObject("Sword");
Sword sword = swordGO.AddComponent<Sword>();
sword.attack_range = 1.5f;
hero.sword = sword;
}
public GameObject FindChild(Transform parent, string childName)
{
GameObject childGO = null;
foreach (Transform child in parent)
{
{
childGO = child.gameObject;
return childGO;
}
else
{
childGO = FindChild(child.transform, childName);
if(childGO != null)
{
return childGO;
}
continue;
}
}
return childGO;
}
// Update is called once per frame
void Update()
{
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
10
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CharacterInfo
{
public int id;
public int hp;
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Character : MonoBehaviour
{
public CharacterInfo info;
public GameObject model;
public Sword sword;
// Start is called before the first frame update
void Start()
{
}
public void SetModel(GameObject model)
{
this.model = model;
if(oldmodel != null)
{
Destroy(oldmodel.gameObject);
}
model.transform.SetParent(this.transform, false);
model.transform.localPosition = Vector3.zero;
}
public void SetInfo(CharacterInfo info)
{
this.info = info;
}
// Update is called once per frame
void Update()
{
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Hero : Character
{
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
10
|
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Monster : Character
{
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
10
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sword : MonoBehaviour
{
public int damage;
public float attack_range;
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
MS API 에는 특정 부모 한정 오브젝트의 이름을 찾기가 없어서 새로운 메소드를 만들어 그것을 구현했다.
'C# > 실습' 카테고리의 다른 글
11.13 unity 유닛 동적생성, 유닛 이동 및 사정거리 내 진입시 공격하기 (0) | 2019.11.13 |
---|---|
11.12 Unity 케릭터 공격모션 + 상대방 피격모션 구현하기 (0) | 2019.11.12 |
11.08 Unity 동적 캐릭터 생성 후 사거리에 따라 공격기능 on/off (0) | 2019.11.08 |
11.07 Unity monster객체에 역직렬화 데이터와 모델 데이터 넣기. (0) | 2019.11.07 |
11.05 Unity 객체, 이벤트, prefeb파일 (2) | 2019.11.05 |