11.29 캐릭터 움직이기 (Coroutine, Update 비교 및 활용)
C#/실습 2019. 11. 29. 16:11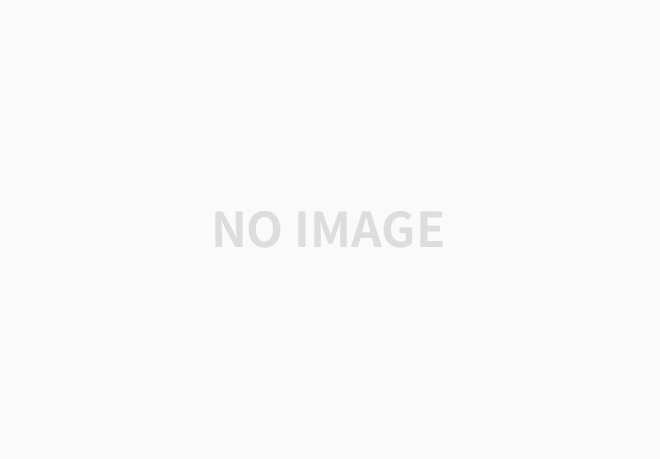
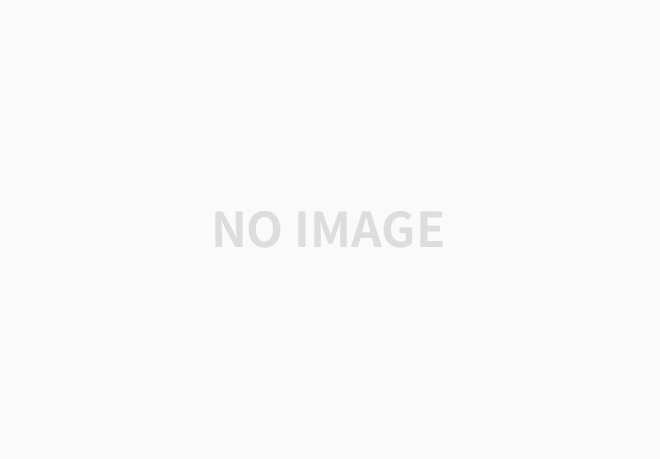
코드:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
using UnityEngine;
using UnityEngine.UI;
public class App : MonoBehaviour
{
public Button btnMoveCoroutine;
public Button btnMoveUpdate;
public Hero hero;
public GameObject targetPoint;
// Start is called before the first frame update
void Start()
{
GameObject heroGO = new GameObject("Hero");
hero = heroGO.AddComponent<Hero>();
string path = string.Format("Prefabs/{0}", "ch_01_01");
var model = Instantiate(prefab);
model.transform.SetParent(heroGO.transform, false);
model.transform.localPosition = Vector3.zero;
hero.model = model;
{
var dis = Vector3.Distance(hero.transform.position, target.transform.position);
var vc = target.transform.position - hero.transform.position;
var dir = vc.normalized;
var speed = 1;
hero.transform.position += dir * speed * Time.deltaTime;
if (dis <= 0.5f)
{
hero.transform.position = target.transform.position;
}
};
btnMoveCoroutine.onClick.AddListener(() =>
{
hero.Move2(targetPoint);
});
btnMoveUpdate.onClick.AddListener(() => {
});
}
// Update is called once per frame
void Update()
{
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System;
public class Hero : MonoBehaviour
{
public bool isMove;
public Action<GameObject> onMove;
public GameObject target;
public GameObject model;
IEnumerator onMoveCoroutine;
// Start is called before the first frame update
void Start()
{
onMoveCoroutine = OnMoveCoroutine();
}
public void Move(GameObject target)
{
this.target = target;
isMove = true;
}
public void Move2(GameObject target)
{
this.target = target;
//isMove = true;
this.model.GetComponent<Animation>().Play("run@loop");
StartCoroutine(onMoveCoroutine);
}
public void Update()
{
if (this.isMove == true)
{
this.model.GetComponent<Animation>().Play("run@loop");
this.onMove(target);
}
if (this.transform.position == target?.transform.position)
{
StopCoroutine(onMoveCoroutine);
}
}
IEnumerator OnMoveCoroutine()
{
while (true)
{
var dis = Vector3.Distance(this.transform.position, target.transform.position);
var vc = target.transform.position - this.transform.position;
var dir = vc.normalized;
var speed = 1;
this.transform.position += dir * speed * 0.01f;
if (dis <= 0.1f)
{
this.model.GetComponent<Animation>().Play("idle@loop");
this.transform.position = target.transform.position;
//isMove = false;
}
yield return new WaitForSeconds(0.01f);
}
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
'C# > 실습' 카테고리의 다른 글
12.06 드래곤플라이트 구현 (0) | 2019.12.06 |
---|---|
12.05 유닛 바라보기 (LookAt 메서드 사용X) (1) | 2019.12.05 |
11.26 Hero와 Monster의 App에 의한 수동적 싸움 (delegate) (0) | 2019.11.26 |
11.13 unity 유닛 동적생성, 유닛 이동 및 사정거리 내 진입시 공격하기 (0) | 2019.11.13 |
11.12 Unity 케릭터 공격모션 + 상대방 피격모션 구현하기 (0) | 2019.11.12 |