10.30 클래스로 LinkedList 구현하기 (메서드, 속성의 재귀호출)
C#/실습 2019. 10. 30. 16:41예제:
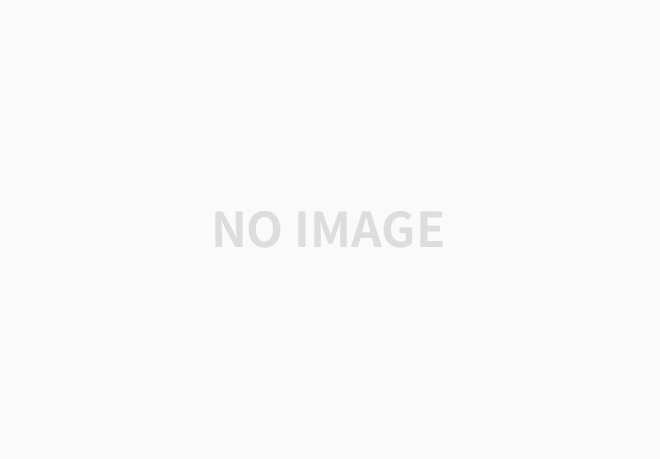
코드:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _10._30_step2
{
class App
{
public App()
{
var list = new LinkedList();
list.Add("홍길동");
list.Add("정약용");
list.Add("이호준");
list.Add("홍길동");
list.Add("정약용");
list.Add("이호준");
Console.WriteLine("인스턴스 개수: {0}",list.Count);
Console.WriteLine("인스턴스 : ");
list.Print();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _10._30_step2
{
class LinkedList
{
public Node first;
public Node node;
public int count = 0;
public int Count
{
get
{
if (first != null)
{
if (node.next != null)
{
node = node.next;
count++;
return Count;
}
else
{
count++;
node = first;
return count;
}
}
return count;
}
}
public LinkedList()
{
Console.WriteLine("LinkedList의 생성자 호출됨");
}
public void Print()
{
if (first != null)
{
if (node.next != null)
{
Console.WriteLine(node.value);
node = node.next;
Print();
}
else
{
Console.WriteLine(node.value);
}
}
}
public void Add(string data)
{
Node node = new Node(data);
if (this.first == null)
{
this.first = node;
this.node = node;
}
else
{
Node lastNode = FindLastNode(this.first);
lastNode.next = node;
}
}
public Node FindLastNode(Node node)
{
if (node.next == null)
{
return node;
}
return FindLastNode(node.next);
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _10._30_step2
{
class Node
{
public string value;
public Node next;
public Node(string data)
{
this.value = data;
Console.WriteLine("새로운 노드 생성됨. (value: {0})", this.value);
}
}
}
여기서 주의해야 할 점은 재귀호출을 할 때이다.
재귀 호출을 한 메소드(반환타입이 있는경우)에서 return문을 사용해 메소드를 종료할 경우, 해당 재귀된 메소드가 종료되는것이지 재귀 호출한 가장 바깥의 메소드가 종료가 되는게 아니기 때문에 if문 다음에 재귀호출 후에 쓰게된 return은 결국 사용하게 된다.
반환 타입이 없는 메소드에서 return을 쓰면 가장 바깥의 메소드를 종료하지만 반환타입이 있는 경우는 for문의 break 와 같이 가장 가까운 메소드를 종료할 뿐이다.
따라서 return (재귀호출문); 을 쓰면 된다.
'C# > 실습' 카테고리의 다른 글
10.31 제너릭을 이용해 사용자 지정 타입 활용하기 (where문 비활용) (0) | 2019.10.31 |
---|---|
10.31 제너릭을 이용해 사용자 지정 타입 활용하기 (where문 활용) (0) | 2019.10.31 |
10.23 Stack 활용하기 (0) | 2019.10.24 |
10.23 배열에서 숫자이동시키기 (0) | 2019.10.24 |
10.24 벡터 (0) | 2019.10.24 |